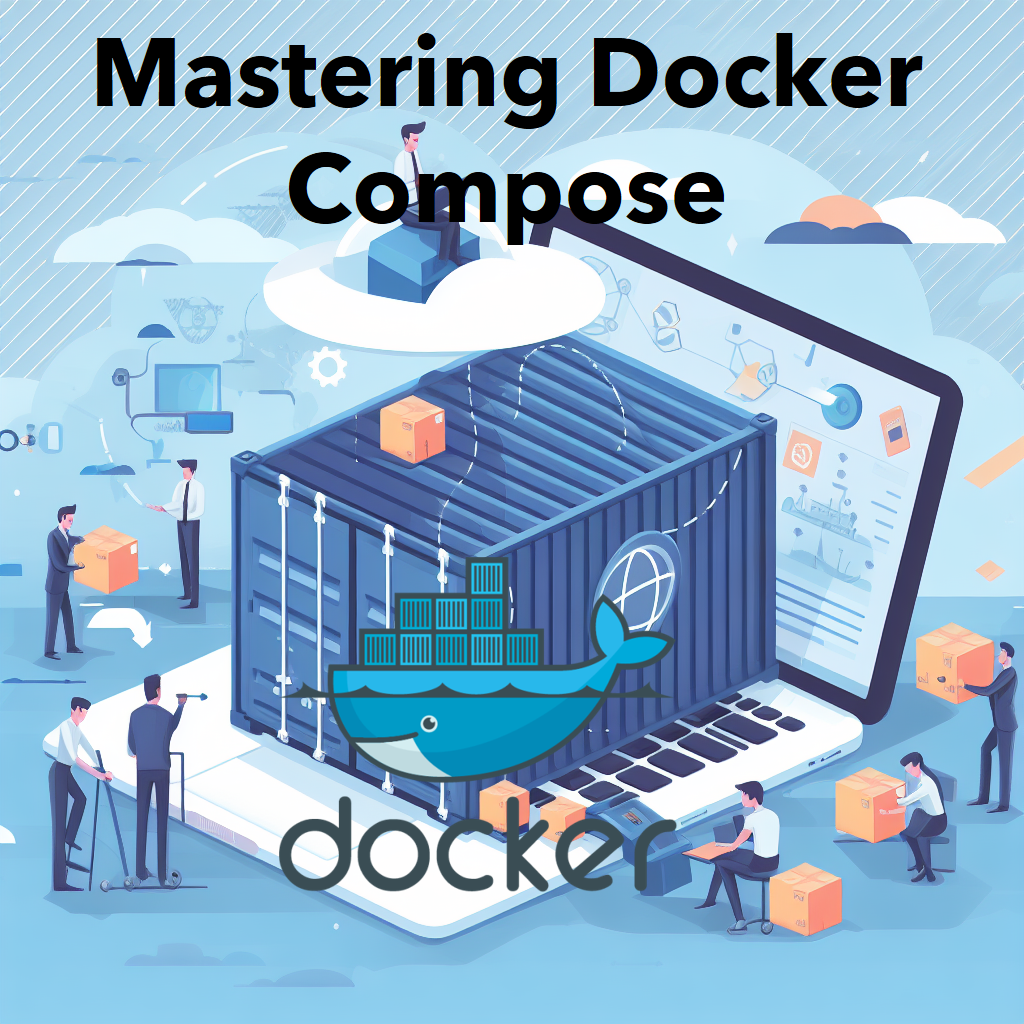
In today’s fast-paced digital world, technology is constantly evolving and finding innovative ways to streamline and optimize processes. One such breakthrough that has revolutionized the world of software development and deployment is containerization. By encapsulating applications and their dependencies in self-contained units, containerization allows for easy and efficient deployment across different environments. When it comes to containerization, Docker is undeniably the king, offering a powerful platform for running and managing containers. But, you may wonder, how can you take your containerization skills to the next level? How can you effectively orchestrate multiple containers, configure networking, and scale your applications effortlessly? Enter Docker Compose, your trusty companion in mastering the art of containerization. In this comprehensive step-by-step guide, we will delve into the ins and outs of Docker Compose and explore how it can help you orchestrate complex multi-container applications with ease and finesse. Whether you’re a seasoned developer looking to level up your Docker skills or a beginner eager to dive into the world of containers, this guide is your roadmap to becoming a containerization maestro. From understanding the fundamentals of Docker Compose to harnessing its powerful features like service definition, network configuration, and volume management, we’ll leave no stone unturned on your journey to containerization mastery. So, grab your coffee, roll up your sleeves, and let’s embark on this exciting adventure together. It’s time to unlock the full potential of Docker Compose and propel your containerized applications to new heights.
The Fundamentals of Docker Compose
- Docker Compose is a tool for defining and running multi-container Docker applications.
- With Compose, you can define a YAML file to define the services and with a single command, you can spin everything up or tear it all down.
- Compose uses the same API used by other Docker commands (CLI commands) and tools.
- Compose file is a YAML file defining services, networks, and volumes for a Docker application.
- Compose supports variables and move a composition between environments.
- Compose can be used for development environments, automated testing environments, and single-host deployments.
- Compose has multiple isolated environments on a single host.
- Compose preserves volume data when containers are created.
- Compose only recreates containers that have changed.
- Compose has the key feature of having a single file that holds the application’s structure and configuration, which makes spinning up applications simple and repeatable everywhere.
Installing Docker Compose
On desktop systems, such as Docker Desktop for Mac and Windows, Docker Compose is already included. No additional steps are needed. On Linux systems, you’ll need to:
- Install the Docker Engine
- Run the following command to download Docker Compose
curl -SL https://github.com/docker/compose/releases/download/v2.24.0/docker-compose-linux-x86_64 -o /usr/local/bin/docker-compose
- Apply permissions to the binary, like so:
sudo ln -s /usr/local/bin/docker-compose /usr/bin/docker-compose
- Test the installation to check it works properly
docker compose version
https://docs.docker.com/compose/install/standalone/
Creating and Managing Services with Docker Compose
A simple example of a Docker Compose file for running WordPress with MySQL using Docker Compose. Save this content in a file named docker compose.yml
version: '3'
services:
# MySQL service
db:
image: mysql:5.7
volumes:
- db_data:/var/lib/mysql
environment:
MYSQL_ROOT_PASSWORD: example_root_password
MYSQL_DATABASE: wordpress
MYSQL_USER: wordpress_user
MYSQL_PASSWORD: example_password
# WordPress service
wordpress:
depends_on:
- db
image: wordpress:latest
ports:
- "8080:80"
volumes:
- wordpress_data:/var/www/html
environment:
WORDPRESS_DB_HOST: db
WORDPRESS_DB_USER: wordpress_user
WORDPRESS_DB_PASSWORD: example_password
WORDPRESS_DB_NAME: wordpress
WORDPRESS_TABLE_PREFIX: wp_
networks:
frontend:
backend:
volumes:
db_data:
wordpress_data:
The provided Docker Compose file (docker-compose.yml
) defines a multi-container setup for running WordPress with a MySQL database. Let’s break down the file:
version: '3'
This specifies the version of the Docker Compose file syntax being used. In this case, it’s version 3.
services: # MySQL service db: image: mysql:5.7 volumes: - db_data:/var/lib/mysql environment: MYSQL_ROOT_PASSWORD: example_root_password MYSQL_DATABASE: wordpress MYSQL_USER: wordpress_user MYSQL_PASSWORD: example_password
This section defines a service named db
using the MySQL 5.7 Docker image. It creates a volume named db_data
to persist MySQL data in the /var/lib/mysql
directory. The environment
section sets environment variables required for MySQL configuration, such as the root password, database name, user, and user password.
# WordPress service wordpress: depends_on: - db image: wordpress:latest ports: - "8080:80" volumes: - wordpress_data:/var/www/html environment: WORDPRESS_DB_HOST: db WORDPRESS_DB_USER: wordpress_user WORDPRESS_DB_PASSWORD: example_password WORDPRESS_DB_NAME: wordpress WORDPRESS_TABLE_PREFIX: wp_
Defining Networks for Multi-Container Applications
You can add a networks
section to your Docker Compose file (docker-compose.yml
). This section allows you to define custom networks that your services will use. Each service can be connected to one or more networks.
version: '3' services: # MySQL service db: image: mysql:5.7 networks: - backend volumes: - db_data:/var/lib/mysql environment: MYSQL_ROOT_PASSWORD: example_root_password MYSQL_DATABASE: wordpress MYSQL_USER: wordpress_user MYSQL_PASSWORD: example_password # WordPress service wordpress: depends_on: - db image: wordpress:latest ports: - "8080:80" networks: - frontend - backend volumes: - wordpress_data:/var/www/html environment: WORDPRESS_DB_HOST: db WORDPRESS_DB_USER: wordpress_user WORDPRESS_DB_PASSWORD: example_password WORDPRESS_DB_NAME: wordpress WORDPRESS_TABLE_PREFIX: wp_ networks: frontend: backend: volumes: db_data: wordpress_data:
- The
db
service is connected to thebackend
network. - The
wordpress
service is connected to bothfrontend
andbackend
networks.
1. Customize Networks:
You can customize the network properties, such as specifying a driver or setting additional options. For example:
networks: frontend: driver: bridge driver_opts: com.docker.network.bridge.name: my_custom_bridge backend: driver: bridge driver_opts: com.docker.network.bridge.name: another_bridge
2. Use Network Aliases:
You can specify network aliases for services. This can be useful when services need to refer to each other by a different name within a specific network.
services: db: networks: backend: aliases: - database wordpress: networks: frontend: aliases: - web
Now, the db
service can be accessed within the backend
network using the alias database
, and the wordpress
service can be accessed within the frontend
network using the alias web
.
3. Connect to Default Network:
If you don’t explicitly define networks, Docker Compose creates a default network for your services. All services in the same docker-compose.yml
file is connected to this default network by default.
By customizing networks, you have more control over how containers communicate in your multi-container applications.
Managing Volumes with Docker Compose
Managing volumes with Docker Compose is crucial for persisting data between container restarts and for sharing data between containers. Docker Compose allows you to define named volumes and bind mounts, providing flexibility for handling data storage. Here’s how you can manage volumes in Docker Compose:
1. Define Volumes in docker-compose.yml
:
You can define named volumes under the volumes
section in your docker-compose.yml
file. Each service can then reference these volumes.
version: "3" services: app: image: your_app_image:latest volumes: - data_volume:/app/data volumes: data_volume:
- The
app
service is using the imageyour_app_image:latest
. - It defines a named volume named
data_volume
and mounts it to the/app/data
the directory inside the container.
2. Bind Mounts:
You can also use bind mounts to mount a directory from the host into the container. This can be useful for development or when you want to control the location of your data on the host machine.
version: '3' services: app: image: your_app_image:latest volumes: - ./local_data:/app/data
- The
app
service mounts the./local_data
directory from the host into the/app/data
the directory inside the container.
3. Volume Drivers and Options:
You can specify volume drivers and options for more advanced configurations.
version: '3' services: app: image: your_app_image:latest volumes: - data_volume:/app/data networks: - my_network volumes: data_volume: driver: local driver_opts: type: none o: bind device: /path/on/host networks: my_network: driver: bridge
- The
data_volume
volume is configured with a local driver and specific options for bind mounting. - The
app
service is connected to themy_network
network.
4. Using External Volumes:
You can use external volumes that are managed outside of Docker Compose.
version: '3' services: app: image: your_app_image:latest volumes: - external_volume:/app/data volumes: external_volume: external: true
- The
app
service uses an external volume namedexternal_volume
.
5. Volume Aliases:
Volume aliases allow you to reference a volume by a different name within a service.
version: '3' services: app: image: your_app_image:latest volumes: - data_volume:/app/data - data_volume:/app/backup:ro volumes: data_volume: driver: local
- The
app
service uses the same volume (data_volume
) twice with different mount points (/app/data
and/app/backup
) and one of them is mounted as read-only (:ro
).
6. Volume Cleanup:
To remove volumes created by the docker-compose up
command, you can use the docker-compose down -v
command.
docker-compose down -v
This will stop and remove the containers and also remove the volumes.
By managing volumes effectively, you ensure data persistence and sharing between containers, making your Dockerized applications more robust and scalable.
Configuring Environment Variables in Docker Compose
Configuring environment variables in Docker Compose is essential for providing configuration to your Dockerized applications. Environment variables are commonly used to store sensitive information, configuration parameters, and other dynamic values. Docker Compose allows you to set environment variables for each service in your docker-compose.yml
file.
Here’s how you can configure environment variables in Docker Compose:
1. Using the environment
key:
You can use the environment
key under each service to set environment variables.
version: '3' services: app: image: your_app_image:latest environment: - DATABASE_URL=mysql://user:password@db_host:3306/database - API_KEY=your_api_key - DEBUG=true
- The
app
service is using the imageyour_app_image:latest
. - The
environment
key is used to define environment variables. - Each environment variable is specified in the format
KEY=VALUE
.
2. Using a .env
File:
You can use a separate .env
file to store your environment variables and then reference that file in your docker-compose.yml
.
.env file:
DATABASE_URL=mysql://user:password@db_host:3306/database API_KEY=your_api_key DEBUG=true
docker-compose.yml:
version: '3' services: app: image: your_app_image:latest env_file: - .env
The env_file
key specifies the path to the .env
file containing your environment variables.
3. Externalizing Environment Variables:
You might want to keep sensitive information separate from your code and Docker Compose files. In such cases, you can use external files or tools to manage your environment variables.
version: '3' services: app: image: your_app_image:latest env_file: - ./config/.env
Here, the environment variables are stored in ./config/.env
. This file could be managed separately and excluded from version control systems.
4. Reference Environment Variables in Docker Compose:
You can reference environment variables directly in your docker-compose.yml
file.
version: '3' services: app: image: your_app_image:latest environment: - DATABASE_URL=${DB_URL}
In this example, the value of DB_URL
will be taken from the environment where you run the docker-compose
command.
5. Using Command Line:
You can override environment variables using the command line when running docker-compose up
.
DATABASE_URL=mysql://user:password@localhost:3306/database docker-compose up
This will override the DATABASE_URL
specifically for this docker-compose up
command.
Configuring environment variables allows you to make your Dockerized applications more flexible and secure by separating configuration from the application code.
Scaling Applications with Docker Compose
Scaling applications with Docker Compose involves running multiple instances of the same service to distribute the workload and handle increased demand. Docker Compose provides a convenient way to scale services easily. Here’s how you can scale applications using Docker Compose:
1. Define a Service to Scale:
In your docker-compose.yml
file, define the service that you want to scale. For example, suppose you have a simple web service:
version: '3' services: web: image: nginx:latest ports: - "8080:80"
In this case, the web
service is running an Nginx web server.
2. Scale the Service:
To scale the service, use the docker-compose up --scale
command, specifying the service name and the desired number of replicas. For example, to run three instances of the web
service:
docker-compose up --scale web=3
This will start three containers running the web
service.
3. Verify Scaling:
You can use the docker-compose ps
command to verify that the specified number of replicas is running:
docker-compose ps
This will show you the status of each container, and you should see three instances of the web
service.
4. Load Balancing:
By default, Docker Compose uses a simple round-robin algorithm for load balancing among scaled service instances. This means that incoming requests are distributed evenly among the running containers.
5. Networking Considerations:
When you scale a service, each instance gets its IP address. However, Docker Compose automatically sets up a common network for the service instances, allowing them to communicate with each other using the service name.
6. Dynamic Port Mapping:
If your service uses dynamic port mapping (e.g., ports: ["8080"]
), each scaled instance will use a different host port. For example, if you scale the web
service to three instances, you might have host ports 8080
, 8081
, and 8082
mapped to the container port 80
.
7. Update or Scale in Real-Time:
You can scale or update services in real time as needed. If you want to scale up or down, simply run the docker-compose up --scale
command with the desired number of replicas.
docker-compose up --scale web=5 # Scale up to 5 replicas docker-compose up --scale web=2 # Scale down to 2 replicas
8. Cleaning Up:
When you’re done testing or need to stop the scaled services, you can use the docker-compose down
command:
docker-compose down
This will stop and remove the containers, networks, and volumes associated with the services.
Scaling with Docker Compose allows you to easily manage the number of instances of your services, making it a convenient tool for handling varying workloads and improving the reliability of your applications.
Deploying and Updating Containerized Applications with Docker Compose
Deploying and updating containerized applications with Docker Compose involves managing the deployment of your services, ensuring high availability, and updating your application when needed. Here’s a step-by-step guide to deploying and updating containerized applications using Docker Compose:
1. Create a Docker Compose File:
Create a docker-compose.yml
file defining your services, networks, volumes, and any necessary configurations. Ensure that your services are configured to meet your application’s requirements.
version: '3' services: web: image: nginx:latest ports: - "8080:80" networks: - my_network networks: my_network:
2. Deploy Your Application:
Use the docker-compose up -d
command to deploy your application in the background:
docker-compose up -d
This command pulls the necessary images and creates and starts the containers, networks, and volumes defined in your docker-compose.yml
file.
3. Verify Deployment:
Check if your containers are running successfully:
docker-compose ps
This will display the status of each service.
4. Scale if Needed:
If your application requires scaling, you can use the docker-compose up --scale
command to scale the services:
docker-compose up --scale web=3
5. Update Your Application:
When you need to update your application, you can do the following:
a. Pull the Latest Images:
docker-compose pull
b. Recreate Containers:
docker-compose up -d --force-recreate
This recreates the containers with the latest images.
6. Rollback:
In case an update causes issues, you can rollback to a previous version:
docker-compose down docker-compose up -d
This will stop and remove the containers and then recreate them based on the original configuration.
7. Clean Up:
When you no longer need the containers or services, you can use the following commands:
docker-compose down
This command stops and removes the containers, networks, and volumes defined in your docker-compose.yml
file.
8. Monitor Logs:
Use docker-compose logs
to monitor logs for your services. This can help troubleshoot during deployment or updates.
docker-compose logs
9. Environment Variables:
If your application requires different configurations for different environments, you can use environment variables in your docker-compose.yml
file or use a .env
file.
10. Security Considerations:
Ensure that you follow security best practices, such as using secure images, limiting unnecessary privileges, and managing sensitive information properly.
11. Continuous Integration/Continuous Deployment (CI/CD):
Consider integrating Docker Compose into your CI/CD pipeline for automated testing and deployment.
Deploying and updating containerized applications with Docker Compose involves a combination of managing configurations, scaling, updating, and monitoring. Regularly review your Docker Compose files and update them as your application evolves.
Best Practices and Tips for Using Docker Compose
Using Docker Compose efficiently involves understanding best practices and adopting strategies that enhance the development, deployment, and maintenance of containerized applications. Here are some best practices and tips for using Docker Compose:
1. Use Version Control:
- Store your
docker-compose.yml
files in version control systems like Git to track changes and manage versions.
2. Environment Variables:
- Use environment variables in your
docker-compose.yml
file to make configurations flexible and easy to manage across different environments.
3. Separate Configuration:
- Externalize sensitive information and configurations using environment files (
.env
files) to keep yourdocker-compose.yml
clean.
4. Named Volumes:
- Use named volumes for persistent data storage. This ensures data persists between container restarts and facilitates easier backup and migration.
5. Container Naming:
- Utilize the
container_name
option to specify explicit container names instead of relying on the default auto-generated names.
6. Scale Services:
- Take advantage of the
docker-compose up --scale
command to easily scale services horizontally based on demand.
7. Use Healthchecks:
- Implement health checks for your services to improve the reliability of your application and enable Docker to monitor the health of containers.
8. Service Dependencies:
- Specify dependencies using
depends_on
to ensure that services start in the correct order, especially when services rely on each other.
9. Keep Containers Stateless:
- Design your containers to be stateless whenever possible. Store persistent data in volumes or external databases to facilitate easier updates and scaling.
10. Optimize Dockerfile:
- Keep your Dockerfiles efficient and follow best practices for image optimization, such as using multi-stage builds to reduce the image size.
11. Cleanup:
- Regularly clean up unused containers, networks, and volumes with commands like `docker-compose down -v` to free up resources.
12. Logging and Monitoring:
- Set up logging and monitoring for your containers. Use `docker-compose logs` for quick troubleshooting, and consider integrating with centralized logging solutions.
13. Network Segmentation:
- Use separate networks for different services to enhance security and provide isolation.
14. Documentation:
- Maintain thorough documentation for your Docker Compose setup, including instructions for deployment, configuration, and updates.
15. CI/CD Integration:
- Integrate Docker Compose into your CI/CD pipeline for automated testing and deployment. Ensure that the CI environment closely resembles the production environment.
16. Update Images Regularly:
- Keep your base images and application images up-to-date to benefit from security patches and new features.
17. Backups:
- Implement backup strategies for your data volumes. Regularly back up critical data to prevent data loss.
18. Version Compatibility:
- Ensure compatibility between Docker Compose versions and the Docker Engine version you are using. Be aware of version-specific features.
19. Community Images:
- When using community images, verify their source and popularity. Stick to well-maintained and trusted images from official repositories.
20. Security Scanning:
- Integrate security scanning tools into your Docker Compose pipeline to identify vulnerabilities in your images.
By following these best practices and tips, you can enhance the reliability, security, and maintainability of your Docker Compose-based applications. Regularly review and update your Docker Compose configurations as your application evolves.
Conclusion: Mastering the Art of Containerization with Docker Compose
Mastering the art of containerization with Docker Compose is a valuable skill that allows developers and operations teams to streamline the deployment, scaling, and management of applications. Docker Compose simplifies the orchestration of multi-container environments, making it easier to develop, test, and deploy applications consistently across different environments.
In conclusion, here are key takeaways for mastering the art of containerization with Docker Compose:
- Understanding Docker Compose:
- Docker Compose simplifies the deployment of multi-container applications by defining services, networks, and volumes in a single YAML file (
docker-compose.yml
). - It allows you to start, stop, and scale your services with a single command, making it efficient for development and testing.
- Docker Compose simplifies the deployment of multi-container applications by defining services, networks, and volumes in a single YAML file (
- Service Definition:
- Clearly define each service in your
docker-compose.yml
file, specifying the image, ports, volumes, and environment variables required for the application.
- Clearly define each service in your
- Networking and Volumes:
- Leverage Docker Compose to define custom networks and named volumes for efficient communication between services and persistent data storage.
- Scaling Applications:
- Use the
docker-compose up --scale
command to easily scale your services horizontally based on demand, ensuring high availability and performance.
- Use the
- Environment Variables:
- Employ environment variables to make your Docker Compose configurations flexible and easily adaptable across different environments.
- Managing Updates:
- Manage application updates by pulling the latest images, recreating containers, and utilizing rollback strategies if needed.
- Security and Best Practices:
- Follow security best practices, externalize sensitive information, and regularly update your Docker Compose configurations.
- Adopt best practices such as using named volumes, health checks, and container naming conventions to enhance reliability and maintainability.
- Continuous Integration and Deployment:
- Integrate Docker Compose into your CI/CD pipeline for automated testing, deployment, and version control.
- Documentation and Cleanup:
- Maintain thorough documentation for your Docker Compose setup, including instructions for deployment, configuration, and updates.
- Regularly clean up unused containers, networks, and volumes to optimize resource usage.
- Community and Collaboration:
- Leverage the Docker community and share your Docker Compose configurations to benefit from collective knowledge and best practices.
By mastering Docker Compose, you empower yourself to efficiently manage complex containerized applications, leading to increased productivity, reliability, and scalability in your software development and deployment processes. Keep exploring new features and staying informed about updates to continuously enhance your skills in the ever-evolving landscape of containerization.